September 15, 2023·ElectronDatabaseselectron-store
electron-store alternatives
We compiled the best alternatives for electron-store that you can use in your next Electron app
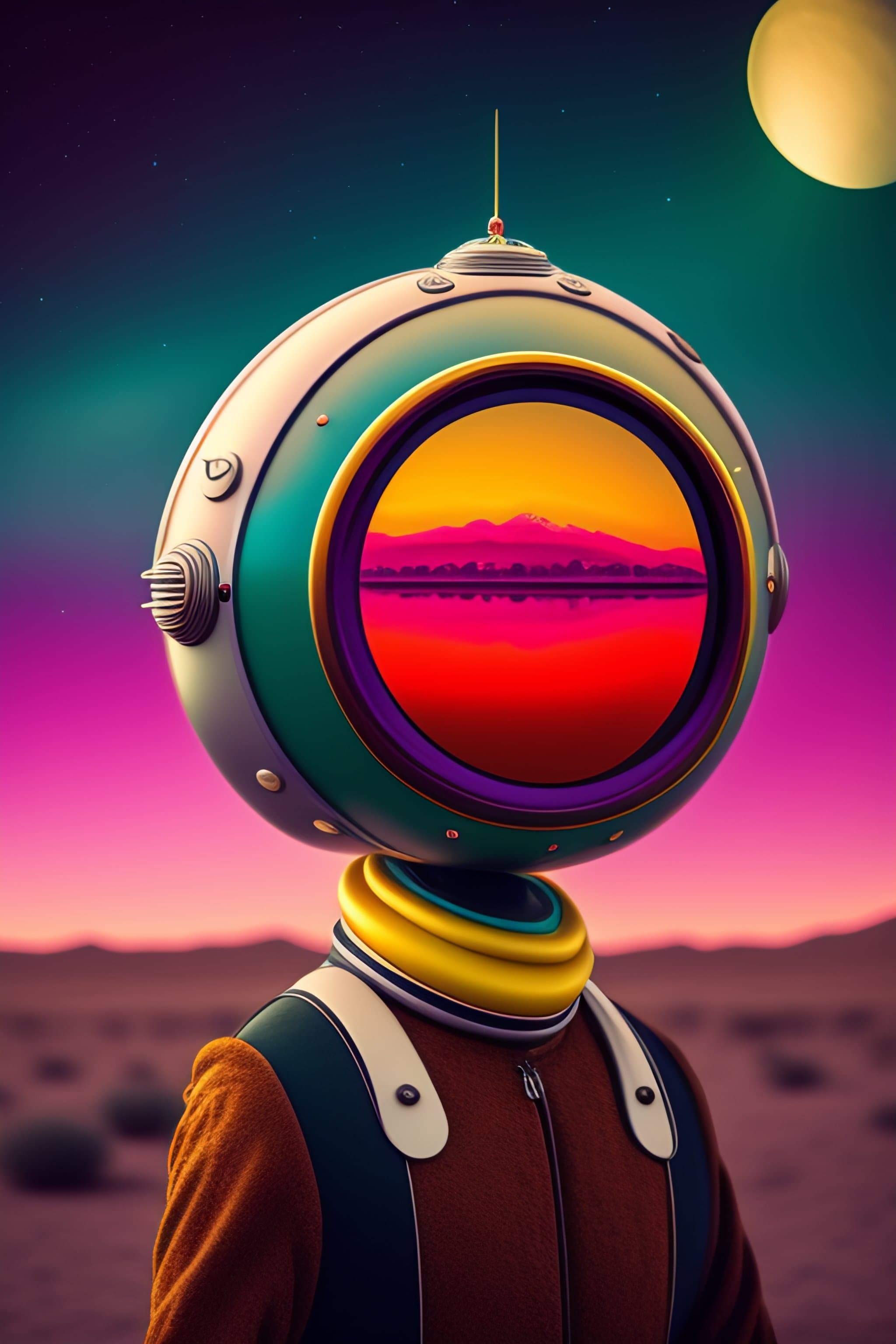
An important aspect of any Electron app is data persistence.
electron-store
was created to fill the gap between a full-fledged database and using window.localStorage
. But it seems like it’s not being maintained anymore, with the last release over a year ago and many open issues.
There’s many alternatives you can use to persist your users data locally:Local Storage / Session Storage
The easiest web-based approach to storing small pieces of data.
localStorage.setItem('key', 'value'); const value = localStorage.getItem('key');
Pros:
- Simple to Use: Local Storage APIs are straightforward and require minimal setup.
- Web Native: Since it's a web-based solution, most front-end developers are already familiar with it.
- No Additional Dependencies: Doesn't require any additional packages to be installed.
Cons:
- Limited Storage: Usually limited to about 5 MB of data.
- Not Secure: Not suitable for storing sensitive information as the data is easily accessible.
- No Advanced Querying: Provides only key-value storage, lacking the ability to perform complex queries.
IndexedDB
A low-level API for client-side storage of significant amounts of structured data, including files/blobs.
const dbRequest = indexedDB.open('myDatabase', 1); dbRequest.onsuccess = (event) => { const db = event.target.result; const transaction = db.transaction(['myStore'], 'readwrite'); const store = transaction.objectStore('myStore'); store.add({key: 'key', value: 'value'}); };
Pros:
- Flexible: Allows storage of large amounts of structured data, including files/blobs.
- Advanced Querying: Supports transactions and various types of queries.
- Web Native: Built into modern web browsers, so no additional dependencies are needed.
Cons:
- Complex API: Has a steep learning curve compared to simpler solutions.
- Browser Differences: While web native, there can be minor differences in implementation across browsers.
- No Built-in Encryption: You'll have to implement encryption yourself if you need to store sensitive data.
SQLite
SQLite provides a full-fledged SQL database for your Electron application. The
sqlite3
npm package can be used for this.const sqlite3 = require('sqlite3').verbose(); const db = new sqlite3.Database('./myDatabase.db'); db.serialize(() => { db.run('CREATE TABLE IF NOT EXISTS key_value (key TEXT, value TEXT)'); db.run('INSERT INTO key_value (key, value) VALUES (?, ?)', ['key', 'value']); });
Pros:
- Robust: Provides a full SQL database engine.
- High Storage Limit: Only limited by the file system.
- ACID Compliance: Supports transactions and rollback capabilities.
Cons:
- Additional Dependency: Requires installing an external package.
- Learning Curve: Requires understanding SQL syntax and database design.
- Platform Differences: Depending on the OS, you might encounter different behavior or performance.
PouchDB / CouchDB
If you want more advanced features like synchronization, PouchDB or CouchDB might suit your needs. PouchDB works well in the browser, and it can sync with CouchDB for server-side storage.
javascriptCopy code const PouchDB = require('pouchdb'); const db = new PouchDB('my_database'); db.put({ _id: 'key', value: 'value' });
Pros:
- Synchronization: Can sync with CouchDB for server-side storage.
- Wide Range of Querying Options: Supports MapReduce queries, among others.
- Web-based: PouchDB is web-native and works well in browser environments.
Cons:
- Additional Dependencies: Requires installation of extra packages.
- Complex Setup for Sync: Implementing real-time synchronization can be complex.
- Performance Overhead: Additional features could lead to performance bottlenecks for simpler applications.
Custom JSON Files
You could read and write JSON files manually to manage application state.
javascriptCopy code const fs = require('fs'); fs.writeFileSync('path/to/file.json', JSON.stringify({ key: 'value' })); const data = JSON.parse(fs.readFileSync('path/to/file.json', 'utf8'));
Pros:
- Easy to Implement: Very straightforward to read and write JSON files using Node.js.
- Portable: Easy to backup, copy, or move.
- No Additional Dependencies: Just use Node.js' built-in
fs
module.
Cons:
- Not Scalable: Reading and writing entire files can become inefficient for large data sets.
- No Querying: You'd have to load data into memory and query it yourself.
DIY
electron-store
is not a very complex package, so there’s always the alternative to write your own module to emulate the functionality.
At its core,
electron-store
reads and writes a JSON file to persist data, so the code would look something like this:
import * as fs from 'fs'; import * as path from 'path'; class SimpleElectronStore { private data: Record<string, any>; private filePath: string; constructor(fileName: string = 'store.json') { const userDataPath = path.resolve('.'); // or app.getPath('userData') this.filePath = path.join(userDataPath, fileName); try { // Try to read the file and parse it as JSON this.data = JSON.parse(fs.readFileSync(this.filePath, 'utf-8')); } catch (error) { // If file read or parse fails, start with an empty object this.data = {}; } } // Get a value from the store get(key: string): any { return this.data[key]; } // Set a value in the store set(key: string, value: any): void { this.data[key] = value; this.save(); } // Delete a value from the store delete(key: string): void { delete this.data[key]; this.save(); } // Save the current state to disk private save(): void { fs.writeFileSync(this.filePath, JSON.stringify(this.data)); } } export default SimpleElectronStore;
We also wrote about the best NoSQL databases for Electron, in case you’re looking for a NoSQL database, though we advise you to think carefully if you prefer NoSQL over SQL.